배너 안에서 풍선이 떨어지는 효과를 자바스크립트를 통해 만들어보자.
배너 닫기 부분 구현 전까지.
풍선이 랜덤으로 내려오는 효과 구현
// 배너
// 이미지 10개
// 사용할 예정
// 사운드를 삽입할 수도 있다. 이번에는 생략
// 필요한 부분들을 객체화
let banner = document.getElementById('banner');
let img = banner.getElementsByTagName('img'); // 베열형식, 동일한 태그를 배열로 가지고 온다
let toggle = document.getElementById('toggle');
// 배너의 높이 가지고 오기
// 높이에 따라 동작이 달라진다
// css 속성값을 가지고 올 수 있다
let banner_height = getComputedStyle(banner).height;
// 풍선을 객체형태로 만들어 관리해주기
// 여러가지 정보를 하나로 묶어서 실제 풍선이 움직일 때 동작을 해야한다.
// 전역변수
let cast = [];
// 객체생성방식
// 이렇게도 할 수 있지만 이번의 경우엔 다른 방식으로 해보겠다.
// let ballInfo = {
// x : x,
// y : y,
// size : size,
// angle : angle,
// speed : speed
// }
// 풍선 객체 생성 함수
// num -> 인덱스 번호
function set_balloon(num){
let x = Math.floor(Math.random() * (500 - 10) + 10); // x축 10 ~ 500
let y = Math.floor(Math.random() * (400 - 120) + 120); // y축 120 ~ 400
let size = Math.floor(Math.random() * (200 - 100) + 100); // 크기 범위 100 ~ 200
let angle = Math.floor(Math.random() * (360 - 0) + 0); // 회전하는 각도 범위 0 ~ 360
let speed = Math.random() * (2 - 0) + 0; // 속도 0 ~ 2
cast[num] = { // cast = [{},{},{},{},... {}]
x : x,
y : -y, // y축은 -일 수록 올라가고, +일 수록 내려간다
size : size,
angle : angle,
speed : speed
}
}
// 각각의 풍선 초기화 함수
function ball_init() {
for (let i = 0; i < img.length; i++) {
// 풍선 객체들을의 속성 초기화
set_balloon(i)
img[i].style.left= '-9999px'; // 풍선의 최초 x좌표 Object.style.css 속성 = "값을 줄 땐 문자열처리"
img[i].style.top = '-9999px'; // 풍선의 최초 y좌표
}
}
function animate_balloon() {
for (let i = 0; i < img.length; i++) {
// 풍선 속성 변경
img[i].style.left = cast[i].x + 'px'; // css 속성 문법에 맞춰 단위를 붙여야 함.
img[i].style.top = cast[i].y + 'px';
img[i].style.transform = 'rotate(' + cast[i].angle + 'deg)'; // 회전각 초기화
if (cast[i].y < parseInt(banner_height)){
// 풍선이 화면에 보임
// 1 ~ 3 하나의 풍선이 가지는 속도
cast[i].y += 1 + cast[i].speed;
cast[i].angle += cast[i].speed;
}else{
// 풍선이 배너 영역을 지나서 화면 밖으로 나갔을 때
set_balloon(i);
}
}
}
ball_init();
// (호출해서 실행하고 싶은 함수, 시간)
setInterval(function(){
animate_balloon();
}, 1000 / 30);
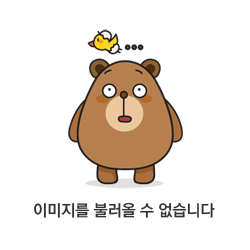
배너 열기, 닫기 기능 추가
toggle.onclick = function(){
// 속성(id, class 등) 중에 class 가 있으면 읽어와라
let attr = banner.getAttribute('class'); // class 속성이 가지고 있는 값을 읽어온다
if (attr === 'active'){
// 베너닫기
banner.removeAttribute('class'); // 해당 속성을 제거
toggle.innerHTML = '배너 열기';
return false; // a 태그에 링크속성 제거
}else {
banner.setAttribute('class', 'active'); // 해당 속성을 추가
toggle.innerHTML = '배너 닫기';
return false;
}
};
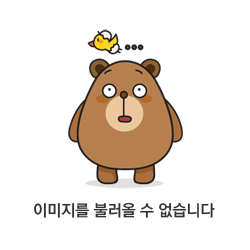

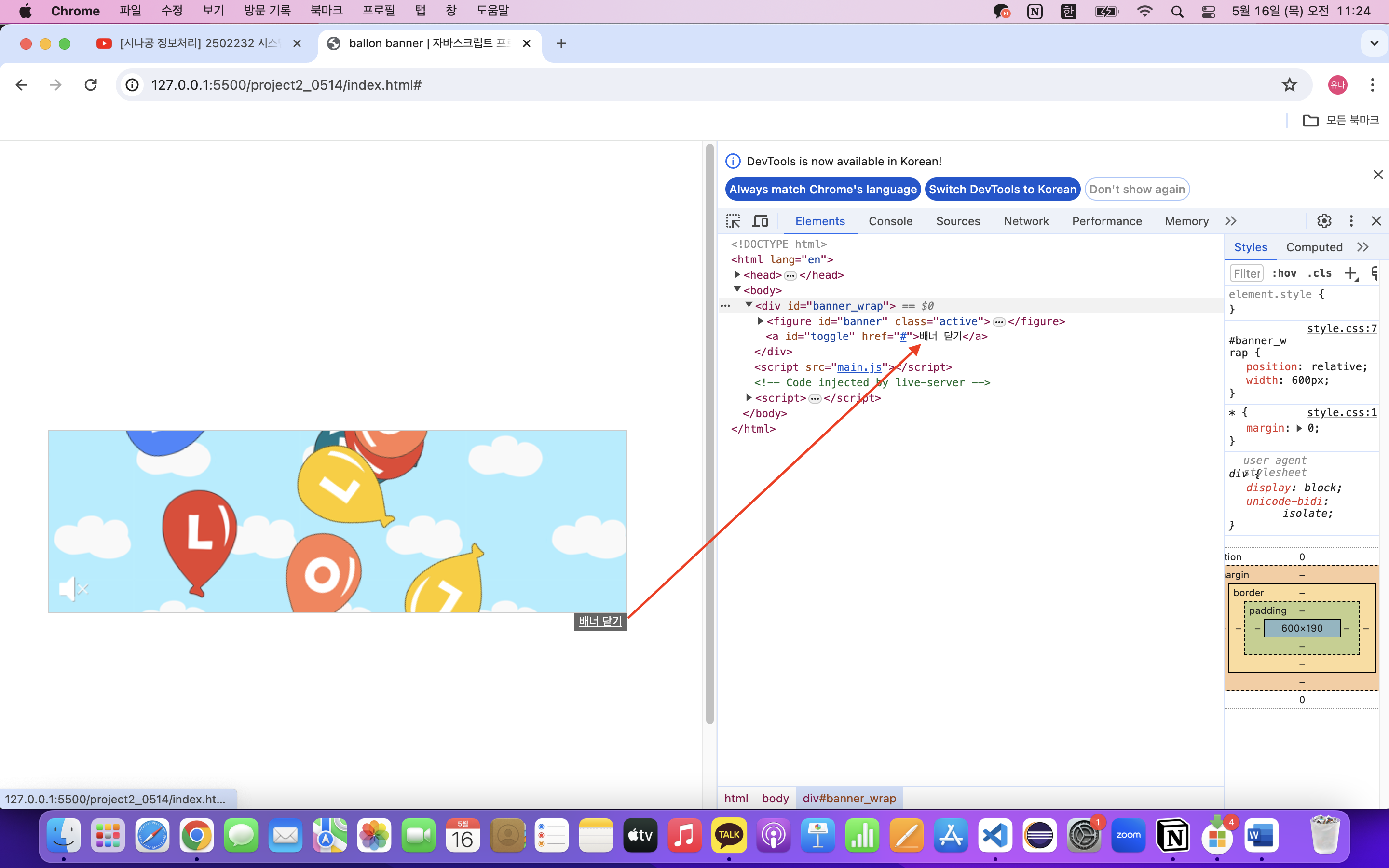
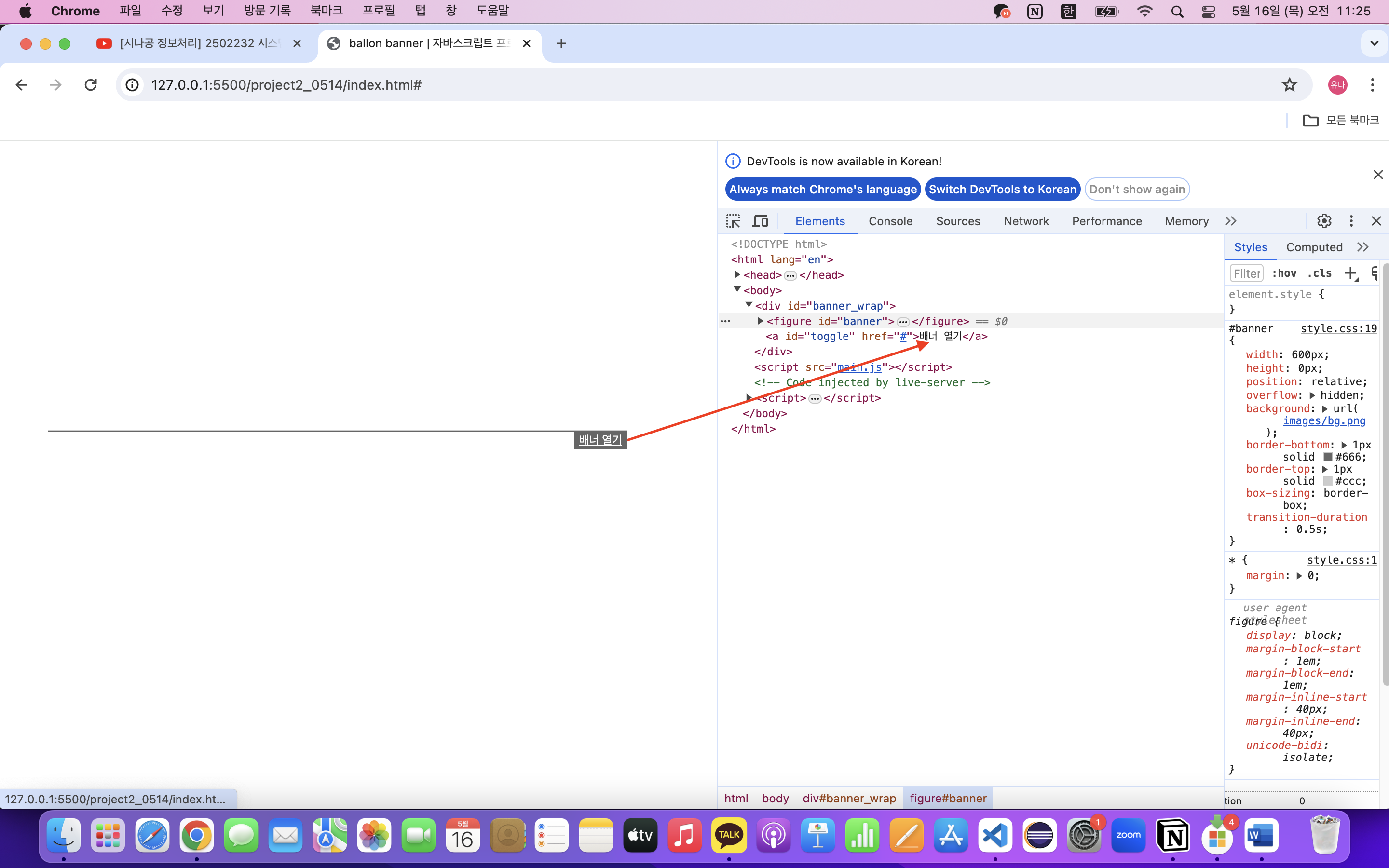
브라우저 콘솔창을 열어보면 배너를 열고 닫았을 때 active 속성이 사라지고, 추가되며 글씨가 바뀌는 것을 알 수 있다.
전체 코드 :
// 배너
// 이미지 10개
// 사용할 예정
// 사운드를 삽입할 수도 있다. 이번에는 생략
// 필요한 부분들을 객체화
let banner = document.getElementById('banner');
let img = banner.getElementsByTagName('img'); // 베열형식, 동일한 태그를 배열로 가지고 온다
let toggle = document.getElementById('toggle');
// 배너의 높이 가지고 오기
// 높이에 따라 동작이 달라진다
// css 속성값을 가지고 올 수 있다
let banner_height = getComputedStyle(banner).height;
// 풍선을 객체형태로 만들어 관리해주기
// 여러가지 정보를 하나로 묶어서 실제 풍선이 움직일 때 동작을 해야한다.
// 전역변수
let cast = [];
// 객체생성방식
// 이렇게도 할 수 있지만 이번의 경우엔 다른 방식으로 해보겠다.
// let ballInfo = {
// x : x,
// y : y,
// size : size,
// angle : angle,
// speed : speed
// }
// 풍선 객체 생성 함수
// num -> 인덱스 번호
function set_balloon(num){
let x = Math.floor(Math.random() * (500 - 10) + 10); // x축 10 ~ 500
let y = Math.floor(Math.random() * (400 - 120) + 120); // y축 120 ~ 400
let size = Math.floor(Math.random() * (200 - 100) + 100); // 크기 범위 100 ~ 200
let angle = Math.floor(Math.random() * (360 - 0) + 0); // 회전하는 각도 범위 0 ~ 360
let speed = Math.random() * (2 - 0) + 0; // 속도 0 ~ 2
cast[num] = { // cast = [{},{},{},{},... {}]
x : x,
y : -y, // y축은 -일 수록 올라가고, +일 수록 내려간다
size : size,
angle : angle,
speed : speed
}
}
// 각각의 풍선 초기화 함수
function ball_init() {
for (let i = 0; i < img.length; i++) {
// 풍선 객체들의 속성 초기화
set_balloon(i)
img[i].style.left= '-9999px'; // 풍선의 최초 x좌표 Object.style.css 속성 = "값을 줄 땐 문자열처리"
img[i].style.top = '-9999px'; // 풍선의 최초 y좌표
}
}
function animate_balloon() {
for (let i = 0; i < img.length; i++) {
// 풍선 속성 변경
img[i].style.left = cast[i].x + 'px'; // css 속성 문법에 맞춰 단위를 붙여야 함.
img[i].style.top = cast[i].y + 'px';
img[i].style.transform = 'rotate(' + cast[i].angle + 'deg)'; // 회전각 초기화
if (cast[i].y < parseInt(banner_height)){
// 풍선이 화면에 보임
// 1 ~ 3 하나의 풍선이 가지는 속도
cast[i].y += 1 + cast[i].speed;
cast[i].angle += cast[i].speed;
}else{
// 풍선이 배너 영역을 지나서 화면 밖으로 나갔을 때
set_balloon(i);
}
}
}
ball_init();
// (호출해서 실행하고 싶은 함수, 시간)
setInterval(function(){
animate_balloon();
}, 1000 / 30);
toggle.onclick = function(){
// 속성(id, class 등) 중에 class 가 있으면 읽어와라
let attr = banner.getAttribute('class'); // class 속성이 가지고 있는 값을 읽어온다
if (attr === 'active'){
// 베너닫기
banner.removeAttribute('class'); // 해당 속성을 제거
toggle.innerHTML = '배너 열기';
return false; // a 태그에 링크속성 제거
}else {
banner.setAttribute('class', 'active'); // 해당 속성을 추가
toggle.innerHTML = '배너 닫기';
return false;
}
};
처음에 배울때는 같은 코드를 안보고 칠 수 있을 정도로 계속 쳐보는 것이 더 좋다.
그러면서 이해하는 것.
강사님이 주는 코드는 최대한 분석해 놓을 것.
이번에는 제이쿼리로 위 코드를 바꿔 볼 것이다.
제이쿼리 ver.
let $banner = $('#banner');
let $img = $banner.find('img');
let $toggle = $('#toggle');
let $banner_height = $banner.css('height');
let cast = [];
function set_balloon(num){
let x = Math.floor(Math.random() * (500 - 10) + 10); // x축 10 ~ 500
let y = Math.floor(Math.random() * (400 - 120) + 120); // y축 120 ~ 400
let size = Math.floor(Math.random() * (200 - 100) + 100); // 크기 범위 100 ~ 200
let angle = Math.floor(Math.random() * (360 - 0) + 0); // 회전하는 각도 범위 0 ~ 360
let speed = Math.random() * (2 - 0) + 0; // 속도 0 ~ 2
cast[num] = { // cast = [{},{},{},{},... {}]
x : x,
y : -y, // y축은 -일 수록 올라가고, +일 수록 내려간다
size : size,
angle : angle,
speed : speed
};
}
function ball_init(){
// each 함수 사용
// 배열 $img 을 가지고 와 하나씩 확인
$img.each(function(i){
set_balloon(i);
$img.eq(i)
.css('left','-9999px')
.css('top', '-9999px')
});
}
function animate_balloon(){
$img.each(function(i){
$img.eq(i)
.css('left', cast[i].x + 'px')
.css('top',cast[i].y + 'px')
.css('transform', 'rotate(' + cast[i].angle + 'deg)');
if (cast[i].y < parseInt($banner_height)){
// 풍선이 화면에 보임
// 1 ~ 3 하나의 풍선이 가지는 속도
cast[i].y += 1 + cast[i].speed;
cast[i].angle += cast[i].speed;
}else{
// 풍선이 배너 영역을 지나서 화면 밖으로 나갔을 때
set_balloon(i);
}
});
}
ball_init();
// (호출해서 실행하고 싶은 함수, 시간)
setInterval(function(){
animate_balloon();
}, 1000 / 30);
문법을 익히기 위해 노력할 것.
스크립트에서 해당 메소드의 이름, 제이쿼리에서 메소드의 이름 이해
이걸 다 할 줄 알아야 진정한 기술탑재 프론트엔드 엔지니어
다음에 볼 시험) 자바스크립트로 프로그램짜기, 그걸 제이쿼리로 바꾸기
해 볼 것 : 계산기 자바스크립트 코드를 제이쿼리 방식으로 바꾸기
계산기 코드 :
// 변수 선언
//document.cal.result // 폼태그를 접근하는 방식 -> 여기서는 아이디나 클래스가 하나하나 선언되어있지 않아 사용 불가
let inp = document.forms['cal'];
let input = inp.getElementsByTagName('input'); // 배열형식으로 값을 가지고 온다
let cls_btn = document.getElementsByClassName('cls_btn')[0]; // 배열형식
let result_btn = document.getElementsByClassName('result_btn')[0]; //배열형식
// 버튼 3그룹
// 1. clear
// 2. 숫자버튼
// 3. 결과버튼
// 계산기 초기화(clear)
function clr(){
inp['result'].value = 0; // 결과창
}
// 계산기 입력 처리 함수
function calc(value){
// 입력이 들어가면 0을 지움
if (inp['result'].value == 0){
inp['result'].value = '';
}
// 입력값을 결과창에 출력
inp['result'].value += value;
}
// 계산 결과 처리 함수
function my_result(){
let result = inp['result'].value;
let cal = eval(result)
//결과창에 표시
inp['result'].value = cal;
}
// 이벤트 핸들러
// -------------------------------------------------------------------
// 숫자 및 사칙연산 버튼
for(var i = 0; i < input.length;i++){
// 숫자와 사칙 연산자만 입력 처리
if(input[i].value != '=' && input[i].value != 'clear'){
input[i].onclick = function(){
calc(this.value); // 숫자의 속성값 의미
};
}
} // end for
// 초기화 버튼
cls_btn.onclick = function(){
clr();
}
// 결과 버튼
result_btn.onclick = function(){
my_result();
}
구현화면 :
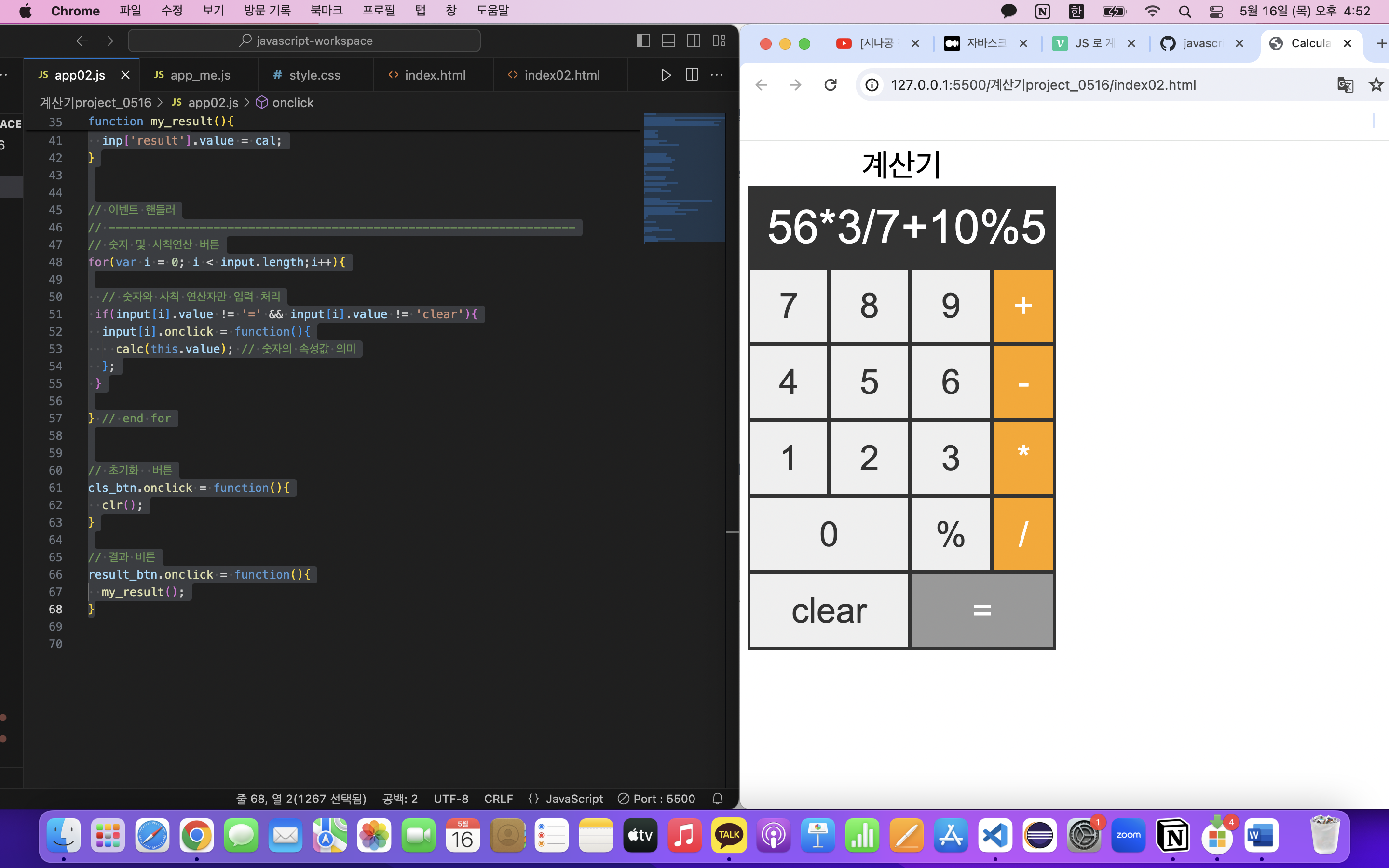
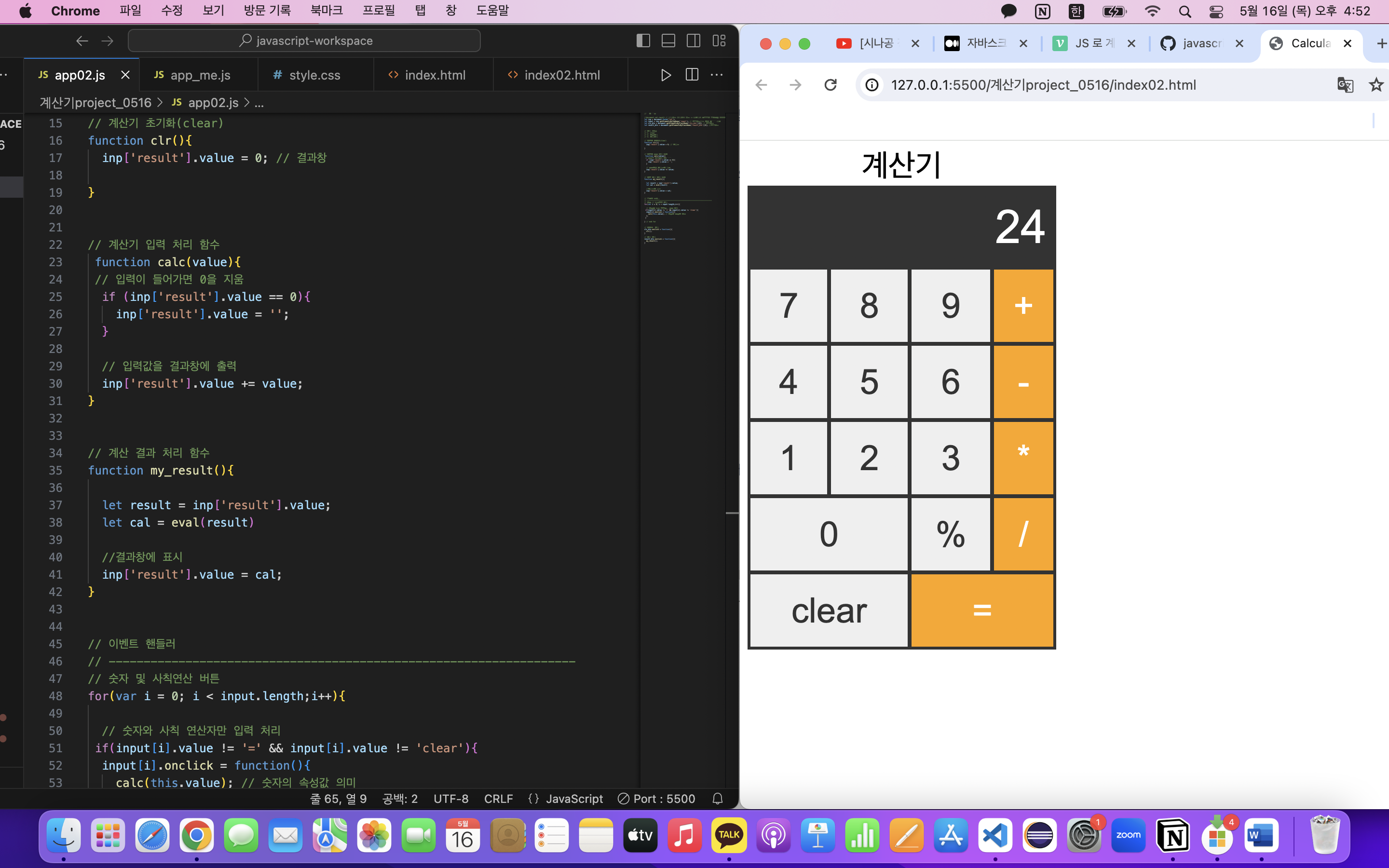
계산이 잘 된다.
하지만 알아보니 eval 함수는 보안 이슈가 있어서 될 수 있으면 쓰지 말아야 한다고.
스스로 풀어볼땐 eval 함수를 쓰지 않는 방법으로 풀어야겠다.
'2024_UIUX 국비 TIL' 카테고리의 다른 글
UIUX _국비과정 0521 [피그마 마무리, 자바2] (1) | 2024.06.11 |
---|---|
UIUX _국비과정 0517~0520 [피그마] (0) | 2024.06.11 |
UIUX _국비과정 0514 [자바스크립트 CBT 구현] (1) | 2024.06.11 |
UIUX _국비과정 0513 [단위평가] (0) | 2024.06.11 |
UIUX _국비과정 0510 [스토리보드를 기반으로 화면구현, 조별과제] (0) | 2024.06.11 |