제이쿼리에 대해 배우고 있는데 메서드들이 많아서 수업시간에 다루는 메서드들은 암기할 필요가 있습니다.
오늘은 웹페이지를 작성할 때 제이쿼리에서 많이 사용되는 것을 배움
→ 객체 조작
제이쿼리의 객체조작
html(), html(요소명)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script
src="https://code.jquery.com/jquery-3.7.1.js"
integrity="sha256-eKhayi8LEQwp4NKxN+CfCh+3qOVUtJn3QNZ0TciWLP4="
crossorigin="anonymous"></script>
<script>
$(function () {
// 객체조작
// 속성조작
// html(),html(요소명) : 특정 태그를 포함한 텍스트까지를 범위로 하는 함수(메서드),(하위요소)
// html() : 해당 대상을 읽어올 때 ()만 쓴다
// html(요소명) : 해당 범위 안에 요소를 추가
let result1 = $("#sec_1").html();
console.log(result1)
$("#sec_1 p").html("<a href=\"#\">Text1</a>");
// text(), text("텍스트") 태그의 텍스트 부분만 범위로 사용한다
let result2 = $("#sec_2").text();
console.log(result2)
$("#sec_2 h2").text("text()");
});
</script>
</head>
<body>
<h1>
<strong>객체 조작 및 생성</strong>
</h1>
<section id="sec_1">
<h2>
<em>html()</em>
</h2>
<p>내용1</p>
</section>
<section id="sec_2">
<h2>
<em>텍스트()</em>
</h2>
<p>내용2</p>
</section>
</body>
</html>
태그의 속성조작
attr("속성명"), attr("속성명", "새로운 값")
removeAttr("속성명") - 삭제
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script
src="https://code.jquery.com/jquery-3.7.1.js"
integrity="sha256-eKhayi8LEQwp4NKxN+CfCh+3qOVUtJn3QNZ0TciWLP4="
crossorigin="anonymous"></script>
<script>
$(function () {
// 태그의 속성 조작
// attr("속성명"), attr("속성명", "새로운 값")
// removeAttr("속성명") - 삭제
let srcVal = $("#sec_1 img").attr("src");
console.log(srcVal);
// 여러개의 속성을 줄 때는 , 로 구분한다
$("#sec_1 img").attr({
"width":200,
"src":srcVal.replace("1.jpg","2.jpg"),
"alt":"바위"
}).removeAttr("border");
});
</script>
</head>
<body>
<h1>
<strong>객체 조작 및 생성</strong>
</h1>
<section id="sec_1">
<h2>이미지 속성</h2>
<p><img src="images/math_img_1.jpg" alt="바위" border="2"></p>
</section>
</body>
</html>
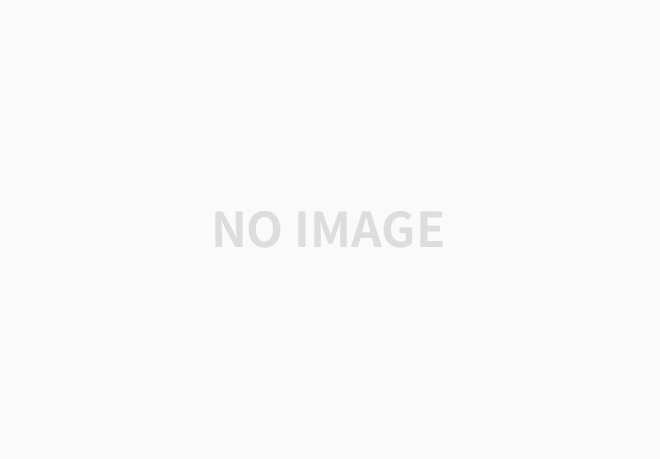
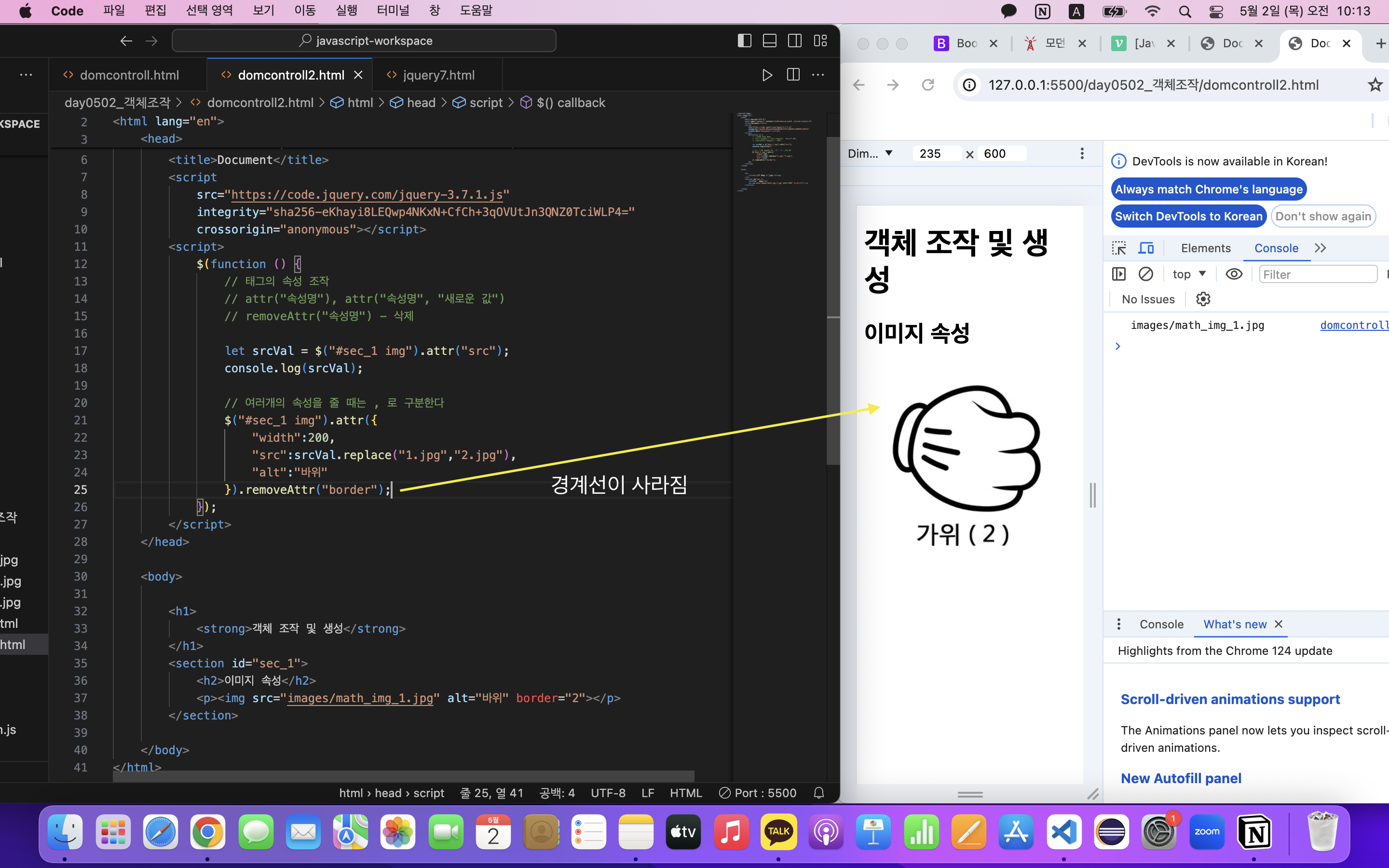
class 속성을 조작하는 메서드
addClass("class값").removeClass("class값")
toggleClass("class값"),hasClass("class값")
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script
src="https://code.jquery.com/jquery-3.7.1.js"
integrity="sha256-eKhayi8LEQwp4NKxN+CfCh+3qOVUtJn3QNZ0TciWLP4="
crossorigin="anonymous"></script>
<script>
$(function () {
// class 속성을 조작하는 메서드
// addClass("class값").removeClass("class값")
// toggleClass("class값"),hasClass("class값")
$("#p1").addClass("aqua");
$("#p2").removeClass("red");
$("#p3").toggleClass("green"); // 없으면 들어가고 있으면 뺀다. -> 상태가 뭐냐에 따라 반대로 작업
$("#p4").toggleClass("green"); // toggle 자체가 '없으면 들어가고 있으면 뺀다' 같은 개념
$("#p6").text($("#p5").hasClass("yellow")); // 값이 있는지 없는지 판단. 있으면 true, 없으면 false
// hasClass() 기존 값이 사라지고 새로운 값이 들어감
});
</script>
<style>
.aqua {
background-color: #0ff;
}
.red {
background-color: #f00;
}
.green {
background-color: #0f0;
}
.yellow {
background-color: #ff0;
}
</style>
</head>
<body>
<p id="p1">내용1</p>
<p id="p2" class="red">내용2</p>
<p id="p3">내용3</p>
<p id="p4" class="green">내용4</p>
<p id="p5" class="yellow">내용5</p>
<p id="p6"></p>
</body>
</html>
폼태그의 값 조작
일반태그일 떄 text()
폼 태그일 땐 val()
폼태그의 값 조작
val(), val("새로운 값")
prop() -> true, false : 선택상자, 체크박스, 라디오버튼 이런 것들을 활용하고 싶을 때
prop() -> 선택되었을 때 true, 선택되지 않았을 때 false , 선택 유무를 알려준다. 상태 체크!
prop("defalutValue")
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script
src="https://code.jquery.com/jquery-3.7.1.js"
integrity="sha256-eKhayi8LEQwp4NKxN+CfCh+3qOVUtJn3QNZ0TciWLP4="
crossorigin="anonymous"></script>
<script>
$(function () {
// 일반태그일 떄 text()
// 폼 태그일 땐 val()
// 폼태그의 값 조작
// val(), val("새로운 값")
// prop() : 선택상자, 체크박스, 라디오버튼 이런 것들을 활용하고 싶을 때
// prop("defalutValue")
let result1 = $("#user_name").val(); // 값을 읽어온다
console.log(result1);
let result2 = $("#user_id").val("javascript"); // () 안의 새로운 값으로 바뀐다
console.log($("#user_id").val());
let result3 = $("#user_id").prop("defaultValue");
console.log(result3);
});
</script>
</head>
<body>
<h1>객체 조작 및 생성</h1>
<form action="#" id="form_1">
<p>
<label for="user_name">이름</label>
<input type="text" name="user_name" id="user_name" value="용대리">
</p>
<p>
<label for="user_id">아이디</label>
<input type="text" name="user_id" id="user_id" value="hello">
</p>
</form>
</body>
</html>
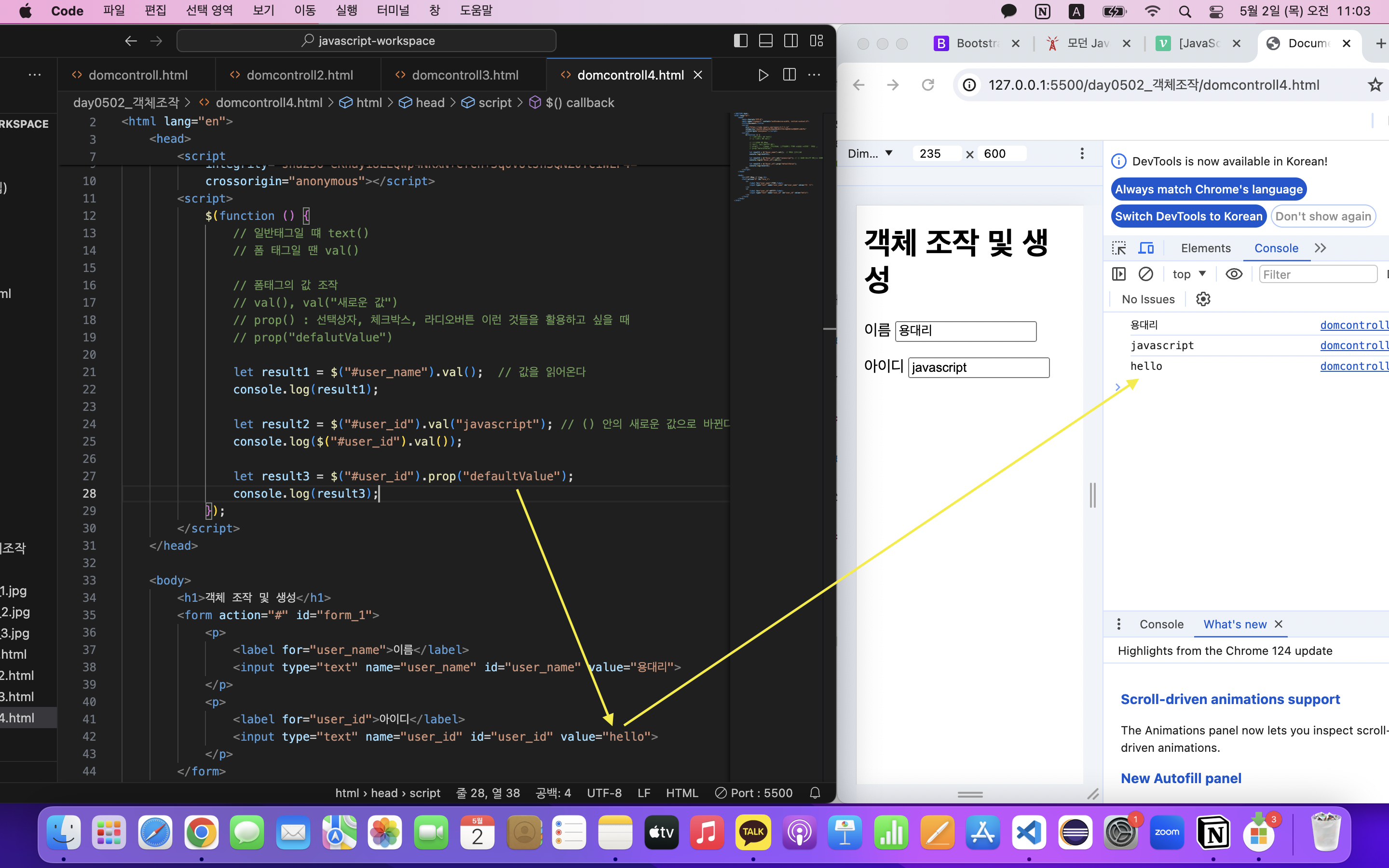
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script
src="https://code.jquery.com/jquery-3.7.1.js"
integrity="sha256-eKhayi8LEQwp4NKxN+CfCh+3qOVUtJn3QNZ0TciWLP4="
crossorigin="anonymous"></script>
<script>
$(function () {
let result1 = $("#chk1").prop("checked");
console.log(result1); // false
let result2 = $("#chk2").prop("checked");
console.log(result2); // true
// 동적처리를 통해 스크립트에서 체크 표시를 변경 가능
let result3 = $("#chk3").prop("checked",true);
// 그룹으로 묶여있을 때
// 부모그룸을 지정하면 하위 그룹을 반환해준다
// selected 되어진 인덱스 번호를 반환해준다.
let result4 = $("#se_1").prop("selectedIndex");
console.log(result4); // 2
});
</script>
</head>
<body>
<h1><strong>객체 조작 및 생성</strong></h1>
<form action="#" id="form_1">
<p>
<input type="checkbox" name="chk1" id="chk1">
<label for="chk1">chk1</label>
<input type="checkbox" name="chk2" id="chk2" checked>
<label for="chk2">chk2</label>
<input type="checkbox" name="chk3" id="chk3">
<label for="chk3">chk3</label>
</p>
<p>
<select name="se_1" id="se_1">
<option value="opt1">option1</option>
<option value="opt2">option2</option>
<option value="opt3" selected>option3</option>
</select>
</p>
</form>
</body>
</html>
수치조작 메서드
객체조작 메서드 ( 수치 조작 메서드 )
객체 조작 메서드 ( 수치 조작 메서드 )
velog.io
width(), height() ....
width("새로운 값"), height("새로운 값") : 크기 변경
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script
src="https://code.jquery.com/jquery-3.7.1.js"
integrity="sha256-eKhayi8LEQwp4NKxN+CfCh+3qOVUtJn3QNZ0TciWLP4="
crossorigin="anonymous"></script>
<script>
$(function () {
// 수치조작 메서드
// width(), height() ....
// width("새로운 값"), height("새로운 값") : 크기 변경
let w1 = $("#p1").height();
console.log(w1); // 50
let w2 = $("#p1").innerHeight();
console.log(w2); // 90
let w3 = $("#p1").outerHeight();
console.log(w3); //100
$("#p2").outerWidth(100).outerHeight(100);
});
</script>
<style>
*{padding: 0;}
#p1, #p2{
width: 100px;
height: 50px;
padding:20px;
border: 5px solid #000;
background-color: #ff0;
}
</style>
</head>
<body>
<h1>수치 조작 메서드</h1>
<p id="p1">내용1</p>
<p id="p2">내용2</p>
</body>
</html>
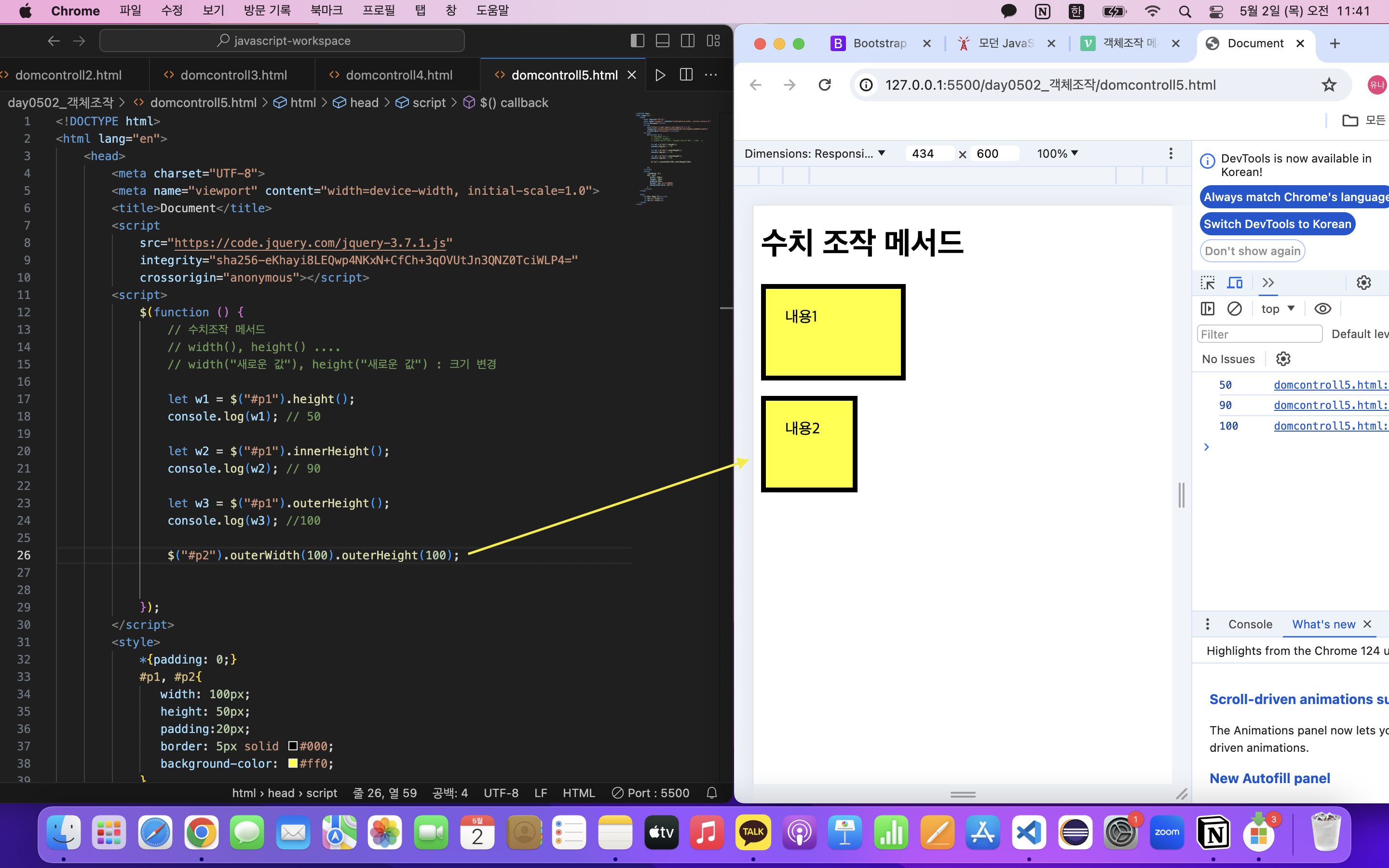
요소의 위치 조작
position().[left, top, right, bottom] : 상대 위치값
offset().[left, top]: 절대 위치값 -> 브라우저로 부터 떨어진 거리
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script
src="https://code.jquery.com/jquery-3.7.1.js"
integrity="sha256-eKhayi8LEQwp4NKxN+CfCh+3qOVUtJn3QNZ0TciWLP4="
crossorigin="anonymous"></script>
<script>
$(function () {
// 요소의 위치 조작
// position().[left, top, right, bottom] : 상대 위치값
// offset().[left, top]: 절대 위치값 -> 브라우저로 부터 떨어진 거리
let $txt1 = $(".txt_1 span"),
$txt2 = $(".txt_2 span"),
$box = $(".box");
let off_t = $box.offset().top; // 100 브라우저가 기준
let pos_t = $box.position().top; // 50 부모, 현재 자신이 기준
$txt1.text(off_t);
$txt2.text(pos_t);
});
</script>
<style>
*{margin:0;padding:0;}
#box_wrap{
width:300px;
height:200px;
margin:50px auto 0;
position: relative;
background-color:#ccc;
}
.box{
width:50px;height:50px;
position:absolute;
left:100px;top:50px;
background-color:#f00;
}
</style>
</head>
<body>
<div id="box_wrap">
<p class="box">박스</p>
</div>
<p class="txt_1">절대 top위칫값: <span></span></p>
<p class="txt_2">상대 top위칫값: <span></span></p>
</body>
</html>
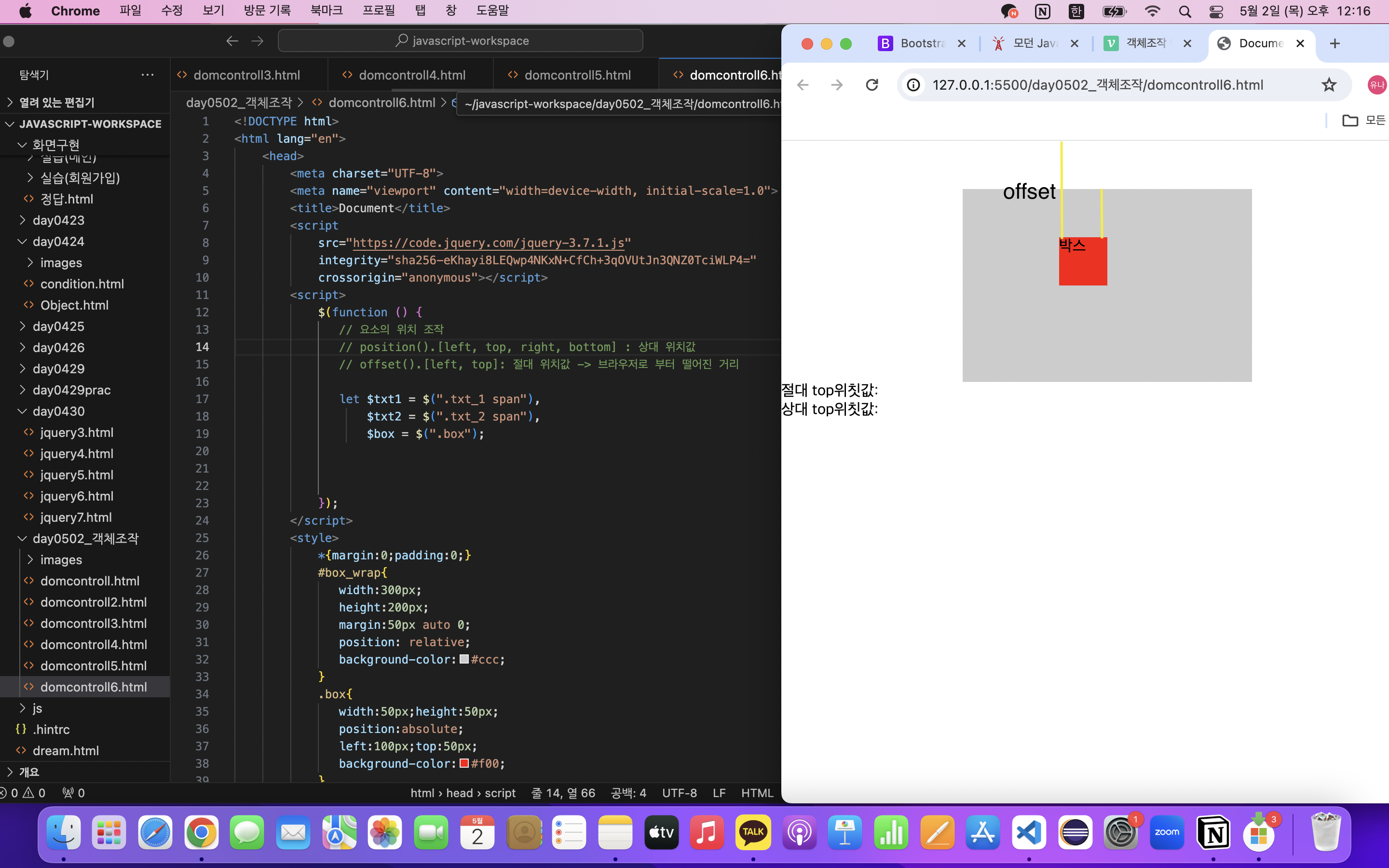
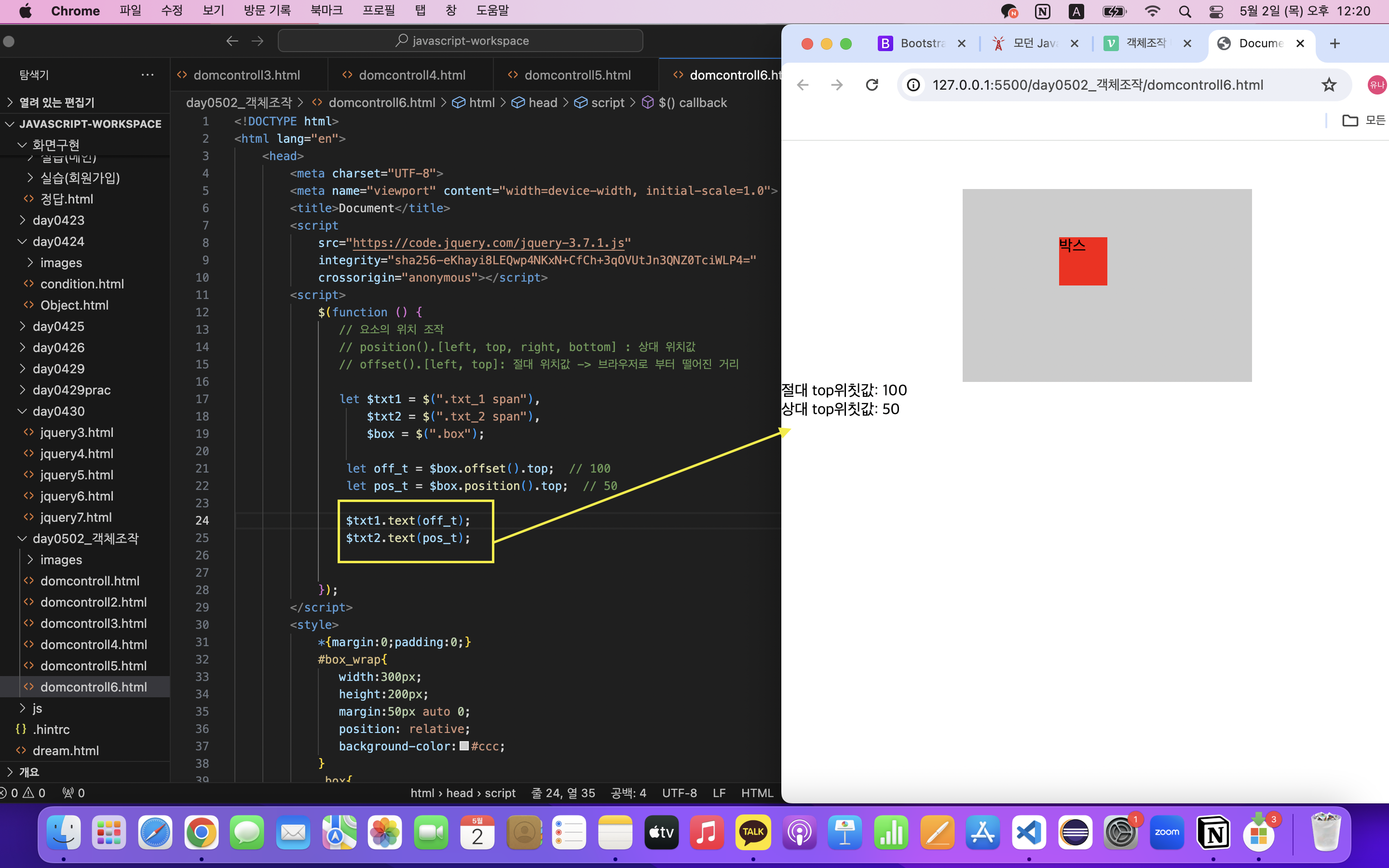
브라우저의 스크롤 수치 조작
scrollTop(), scrollLeft() : 수치를 읽어올 때
scrollTop(새로운 값), scrollLeft(새로운 값)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script
src="https://code.jquery.com/jquery-3.7.1.js"
integrity="sha256-eKhayi8LEQwp4NKxN+CfCh+3qOVUtJn3QNZ0TciWLP4="
crossorigin="anonymous"></script>
<script>
$(function () {
// 브라우져의 스크롤 수치 조작
// scrollTop(), scrollLeft() : 수치를 읽어올 때
// scrollTop(새로운 값), scrollLeft(새로운 값)
// let topNum = $("h1").offset().top;
// console.log(topNum); // 2000px
// $(window).scrollTop(topNum);
// let sct = $(window).scrollTop();
// console.log(sct); // 2000px 만큼 위치 이동
$(window).scroll(function(){
let sct = $(window).scrollTop();
console.log(sct);
if(sct <= 2000){
$("body").css({"background-color":"tomato"});
}else if(sct > 2000 && sct <= 3000){
$("body").css({"background-color":"orange"});
}else{
$("body").css({"background-color":"yellow"});
}
});
});
</script>
<style>
*{
margin:0;
padding:0;
}
body{
line-height:1;
}
#wrap{
height:5000px;
padding-top:2000px;
}
</style>
</head>
<body>
<div id="wrap">
<h1>위치 메서드</h1>
</div>
</body>
</html>
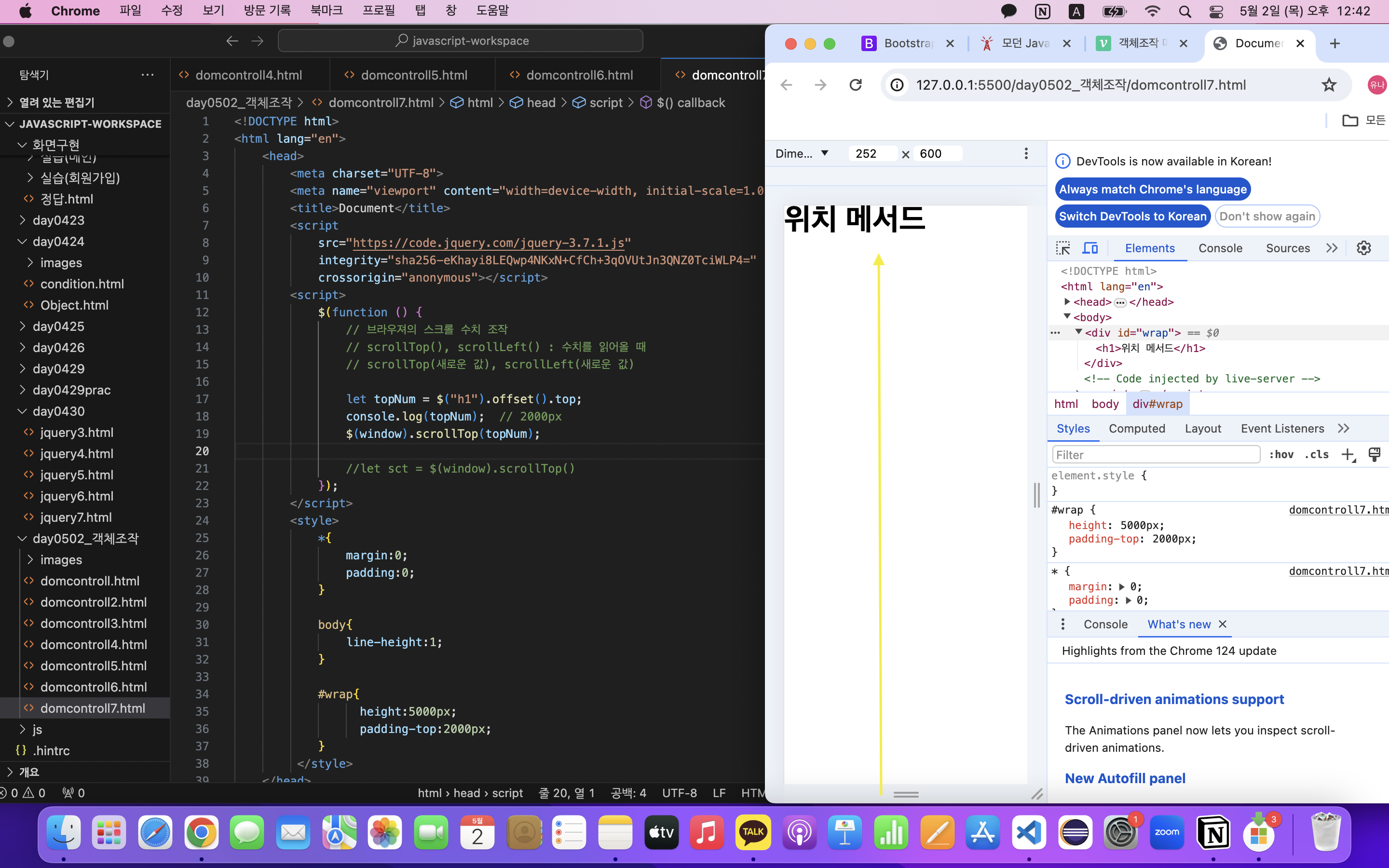
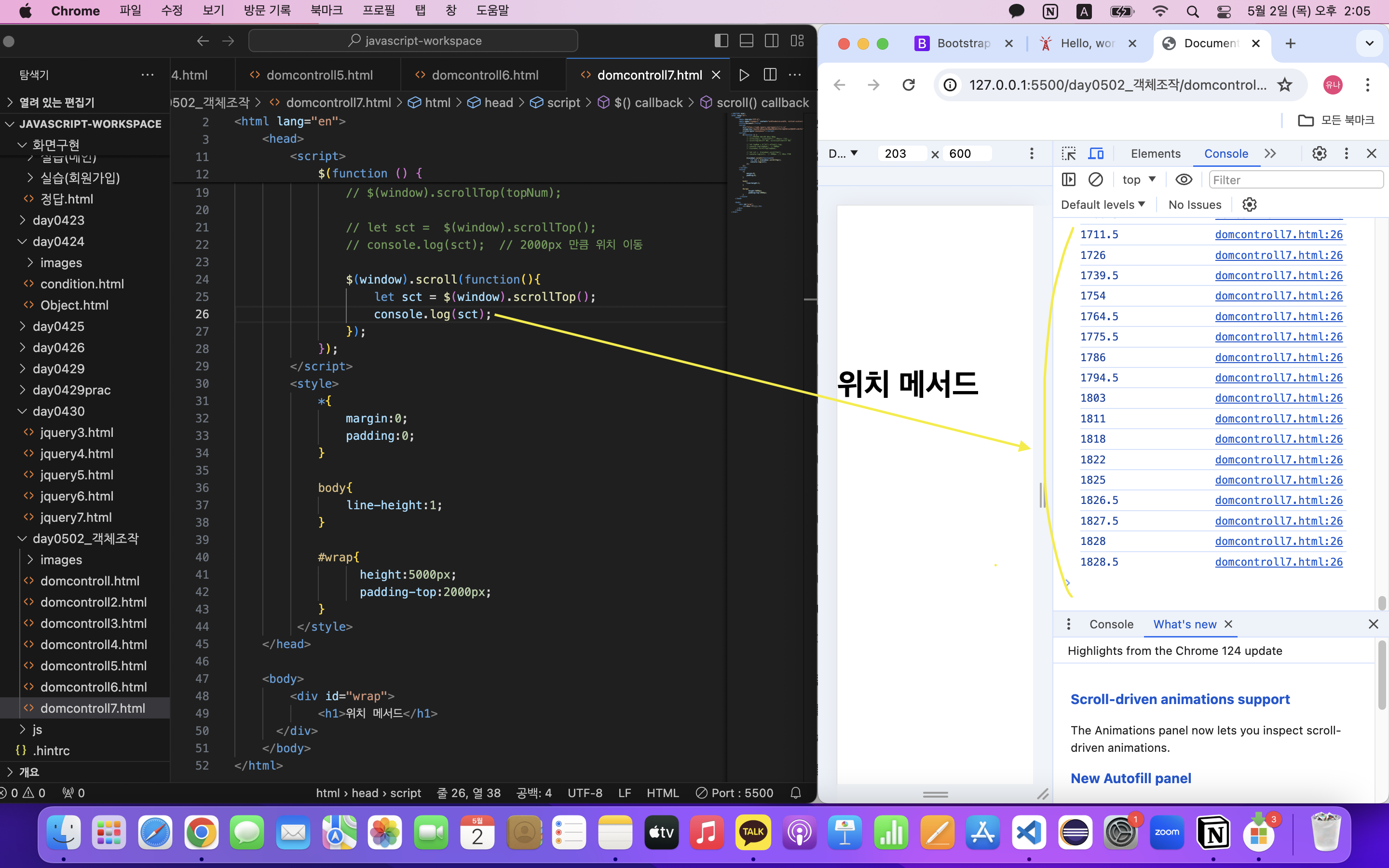
객체 요소 편집
생성
before(), after(),
append(), appendTo(),
prepend(), prependTo(),
insertBefore(), insertAfter()
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script
src="https://code.jquery.com/jquery-3.7.1.js"
integrity="sha256-eKhayi8LEQwp4NKxN+CfCh+3qOVUtJn3QNZ0TciWLP4="
crossorigin="anonymous"></script>
<script>
$(function () {
// 객체 요소 편집
// 생성, ...
// before(), after(),
// append(), appendTo(),
// prepend(), prependTo(),
// insertBefore(), insertAfter()
// remove()
// 동위
// $("#wrap p:eq(2)").after("<p>After</p>"); // 기준을 앞에 정의
// $("<p>insertAfter</p>").insertAfter("#wrap p:eq(1)"); // 기준을 뒤에 정의
// $("#wrap p:eq(1)").before("<p>Before</p>");
// $("<p>insertBefore</p>").insertBefore("#wrap p:eq(0)");
// 하위
$("<li>appendTo</li>").appendTo("#listZone"); // 가장 마지막에 위치한다
$("#listZone").prepend("<li>prepend</li>"); // 가장 앞쪽에 위치한다.
});
</script>
</head>
<body>
<ul id="listZone">
<li>리스트</li>
</ul>
<!-- <div id="wrap">
<p>내용1</p>
<p>내용2</p>
<p>내용3</p>
</div> -->
</body>
</html>
복제, 삭제 ...
clone() : 복제
remove() : 삭제(해당요소를 포함한 모두 삭제)
empty() : 삭제(해당요소의 하위 요소만 삭제, 해당요소 자체는 남겨둔다.)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script
src="https://code.jquery.com/jquery-3.7.1.js"
integrity="sha256-eKhayi8LEQwp4NKxN+CfCh+3qOVUtJn3QNZ0TciWLP4="
crossorigin="anonymous"></script>
<script>
$(function () {
// 객체 요소 편집
// 복제, 삭제 ...
// clone() : 복제
// remove() : 삭제(해당요소를 포함한 모두 삭제)
// empty() : 삭제(해당요소의 하위 요소만 삭제, 해당요소 자체는 남겨둔다.)
// children() 자식요소들을 가지고 옴
let copyObj = $(".box1").children().clone();
$(".box2").remove();
$(".box3").empty();
$(".box3").append(copyObj);
});
</script>
</head>
<body>
<div class="box1">
<p>내용1</p>
<p>내용2</p>
</div>
<div class="box2">
<p>내용3</p>
<p>내용4</p>
</div>
<div class="box3">
<p>내용5</p>
<p>내용6</p>
</div>
</body>
</html>
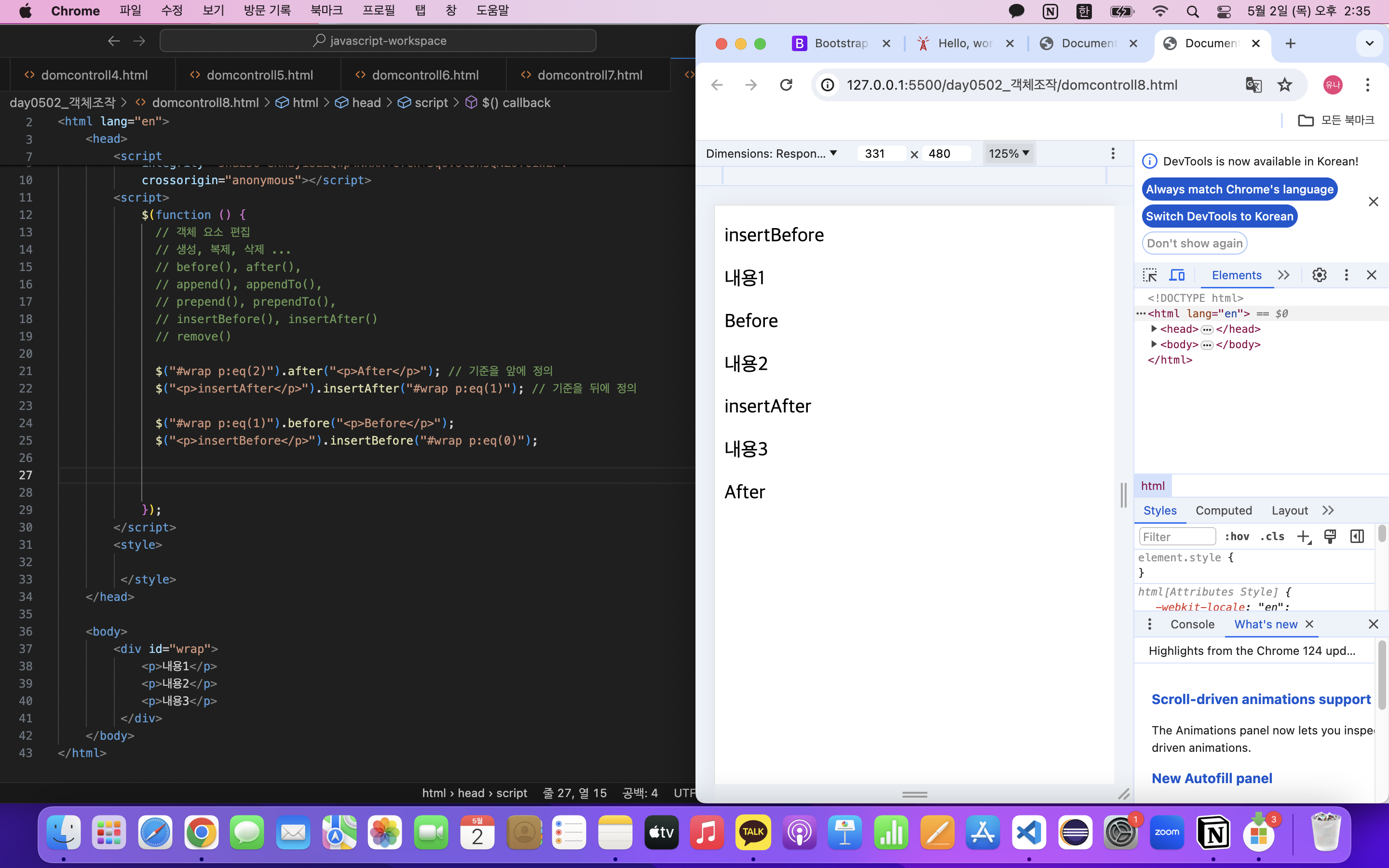
제이쿼리의 이벤트
참고)
[jQuery]이벤트(Event) 종류
⊙ 이벤트(Event) •기본적으로 전역에 작성된 프로그램은 프로그램이 실행됨과 동시에 바로 실행되...
blog.naver.com
제이쿼리 이벤트를 주는 두 가지 방식
mouseover, mouseout, focus, blur
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script
src="https://code.jquery.com/jquery-3.7.1.js"
integrity="sha256-eKhayi8LEQwp4NKxN+CfCh+3qOVUtJn3QNZ0TciWLP4="
crossorigin="anonymous"></script>
<script>
$(function () {
// 제이쿼리 이벤트를 주는 두 가지 방식
// 단독 이벤트, 그룹 이벤트
// 단독 이벤트
// $("선택자").이벤트(익명함수 방식의 핸들러 function(){});
// 그룹 이벤트 만드는 방식 두 가지.
// 이벤트 나열할때 스페이스로 구분
// $("선택자").on("이벤트종류1 이벤트종류2 이벤트종류n" , 익명함수 방식의 핸들러 function(){});
// $("선택자").on({"이벤트종류1 이벤트종류2 이벤트종류n":function(){
// }
// });
$(".btn1").click(function(){
$(".btn1").parent().next().css({"color":"red"});
});
// mouseover 마우스를 해당요소에 올려놓았을 때
// mouseout 마우스를 해당 요소에서 다른 곳에 두었을 때
// focus 노드가 포커스를 획득했을 때
// blur 포커스가 해당 요소를 벗어날때 -> tap으로 실행해볼 수 있음
$(".btn2").on({
"mouseover focus":function(){
$(".btn2").parent().next().css({"color":"red"});
},
"mouseout blur":function(){
$(".btn2").parent().next().css({"color":"blue"});
}
});
// 이벤트 제거(이벤트를 동결시킬 때 사용)
$(".btn1").off("click");
});
</script>
</head>
<body>
<p>
<button class="btn1">버튼1</button>
</p>
<p>내용1</p>
<p>
<button class="btn2">버튼2</button>
</p>
<p>내용2</p>
</body>
</html>
hover
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script
src="https://code.jquery.com/jquery-3.7.1.js"
integrity="sha256-eKhayi8LEQwp4NKxN+CfCh+3qOVUtJn3QNZ0TciWLP4="
crossorigin="anonymous"></script>
<script>
$(function () {
// mouseover 마우스를 해당요소에 올려놓았을 때
// mouseout 마우스를 해당 요소에서 다른 곳에 두었을 때
// mouseover와 mouseout 사용
$(".btn1").on({
"mouseover":function(){
$(".txt1").css({"background-color":"yellow"});
},
"mouseout":function(){
$(".txt1").css({"background":"none"});
}
});
// mouseover와 mouseout를 같이 처리해주는 hover
// hover => mouseover + mouseout
// hover는 focus와 같은 그륩이벤트 적용이 안된다.
$(".btn2").hover(
function(){ // mouseover
$(".txt2").css({"background-color":"skyblue"});
},
function(){ // mouseout
$(".txt2").css({"background":"none"});
}
);
});
</script>
</head>
<body>
<p><button class="btn1">Mouse Over/Mouse Out</button></p>
<p class="txt1">내용1</p>
<p><button class="btn2">Hover</button></p>
<p class="txt2">내용2</p>
</body>
</html>
mousemove 이벤트
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script
src="https://code.jquery.com/jquery-3.7.1.js"
integrity="sha256-eKhayi8LEQwp4NKxN+CfCh+3qOVUtJn3QNZ0TciWLP4="
crossorigin="anonymous"></script>
<script>
// mousemove
// mouse가 움직이는 좌표값을 받아서 이벤트를 발생
// function(e) -> e 는 event 객체, X축과 Y축의 값을 받아올 수 있다
// 키보드와 마우스는 운영체제가 관리
// 윈도우를 띄웠을 때 보이는 흰색 배경을 document라고 한다.
$(function () {
$(document).on("mousemove",function(e){
$(".posX").text(e.pageX);
$(".posY").text(e.pageY);
});
});
</script>
</head>
<body>
<h1>mousemove</h1>
<p>X : <span class="posX">0</span>px</p>
<p>Y : <span class="posY">0</span>px</p>
</body>
</html>
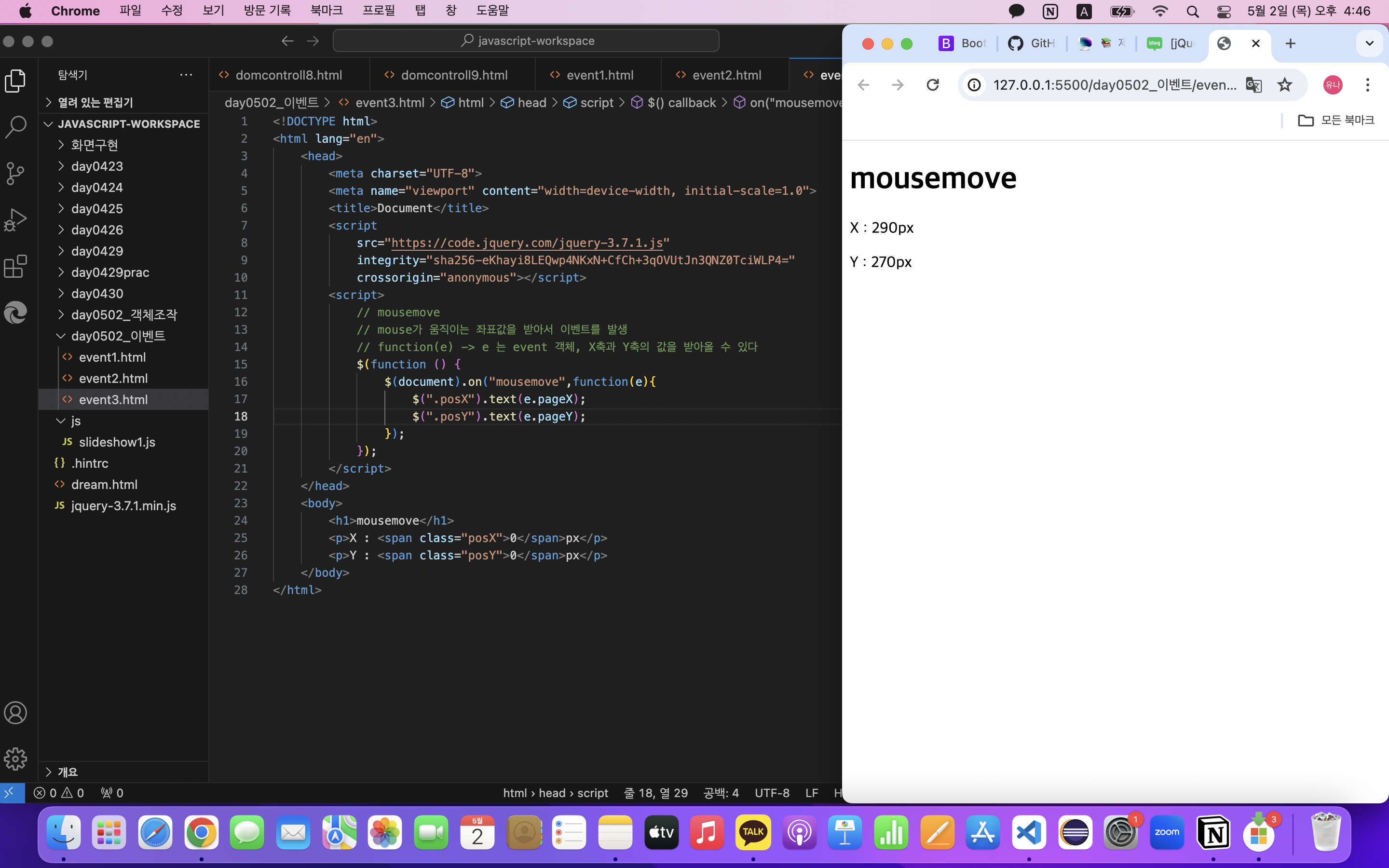
'2024_UIUX 국비 TIL' 카테고리의 다른 글
UIUX _국비과정 0507 [파워포인트 목업] (1) | 2024.06.08 |
---|---|
UIUX _국비과정 0503 [제이쿼리 이벤트] (1) | 2024.06.08 |
UIUX _국비과정 0430 [제이쿼리 선택자] (1) | 2024.06.08 |
UIUX _국비과정 0429 [제이쿼리 입문] (1) | 2024.06.04 |
UIUX _국비과정 0426 (0) | 2024.06.03 |