▼ applicationContext
이전까지 작업했던 내용 )
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.3.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.3.xsd">
<!-- 패키지를 스캔해준다. 다 해주는 것은 아니고 표시되어진 클래스만 스캔해준다 -> 이것이 어노테이션 -->
<!-- <context:component-scan base-package="polymorphism"></context:component-scan> -->
<context:component-scan base-package="com.springbook.biz"></context:component-scan>
<!-- <bean id="log" class="com.springbook.biz.common.LogAdvice" ></bean> -->
<bean id="log" class="com.springbook.biz.common.Log4jAdvice" ></bean>
<bean id="around" class="com.springbook.biz.common.AroundAdvice" ></bean>
<aop:config>
<aop:pointcut expression="execution(* com.springbook.biz..*Impl.*(..))" id="allPointcut"/>
<aop:aspect ref="around"> <!-- 동작시점 -->
<!-- 대상, 적용할 기능 -->
<aop:around pointcut-ref="allPointcut" method="aroundLog"/>
</aop:aspect>
</aop:config>
<!-- = SamsungTV tv = new SamsungTV(); -->
<!-- <bean id="" class=""></bean> -->
<!-- <bean id="tv" class="polymorphism.SamsungTV"></bean> 싱글톤 패턴 사용 -->
<!-- scope="prototype"을 추가하면 싱글톤 패턴 사용을 하지 않을 수 있다. -->
<!-- 생성자 의존 주입 -->
<!--<bean id="tv" class="polymorphism.SamsungTV" scope="prototype"> -->
<!-- 괸계설정 -->
<!-- <constructor-arg ref="apple"></constructor-arg> -->
<!-- 기본형 관계설정 시 value를 쓴다 -->
<!-- <constructor-arg value="10000"></constructor-arg> -->
<!-- </bean> -->
<!-- Setter 메소드 의존 주입 -->
<!-- <bean id="tv" class="polymorphism.SamsungTV" scope="prototype">
<property name="speaker" ref="sony"></property>
<property name="price" value="10000"></property>
</bean>
<bean id="sony" class="polymorphism.SonySpeaker"></bean>
<bean id="apple" class="polymorphism.AppleSpeaker"></bean> -->
</beans>
스프링의 3대 요소
AOP (Aspect Oriented Programmig)
: 관심지향 (공통 관심사)
로깅, 보안, 트랜잭션 관리 등과 같은 공통적인 관심사를 모듈화 해 코드 중복을 줄이고 유지 보수성을 형성하는데 도움을 준다.
공통관심사는 대상기능의 실행 전, 후로 나뉜다.
로그에 대해 이해하기
LogAdvice.java를 수행할 땐 Log4jAdvice.java 메소드를 주석 처리,
Log4jAdvice.java를 실행할 땐 LogAdvice.java 메소드를 주석 처리해야한다.
LogAdvice.java
package com.springbook.biz.common;
// --------------- 공통관심사 ---------------
public class LogAdvice {
public void printLog() {
System.out.println("[공통로그] 비즈니스 로직 수행 전");
}
}
// --------------------------------------
Log4jAdvice.java 심화된 공통 관심사
package com.springbook.biz.common;
public class Log4jAdvice {
public void printLogging() {
System.out.println("[공통로그 - Log4j] 비즈니스 로직 수행 전 동작");
}
}
BoardServiceImpl.java
package com.springbook.biz.board.impl;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.springbook.biz.board.BoardService;
import com.springbook.biz.board.BoardVO;
import com.springbook.biz.common.Log4jAdvice;
import com.springbook.biz.common.LogAdvice;
@Service("boardService")
public class BoardServiceImpl implements BoardService {
// boardDAO를 의존 주입해야 한다.
@Autowired
private BoardDAO boardDAO;
private Log4jAdvice log;
public BoardServiceImpl() {
log = new Log4jAdvice();
}
// private LogAdvice log;
//
// public BoardServiceImpl() {
// log = new LogAdvice();
// }
@Override
public void insertBoard(BoardVO vo) {
// @Autowired를 했기 때문에 사용가능, @Autowired로 객체를 생성한거다.
//log.printLog();
log.printLogging();
boardDAO.insertBoard(vo);
}
@Override
public void updateBoard(BoardVO vo) {
//log.printLog();
log.printLogging();
boardDAO.updateBoard(vo);
}
@Override
public void deleteBoard(BoardVO vo) {
//log.printLog();
log.printLogging();
boardDAO.deleteBoard(vo);
}
@Override
public BoardVO getBoard(BoardVO vo) {
//log.printLog();
log.printLogging();
return boardDAO.getBoard(vo);
}
@Override
public List<BoardVO> getBoardList(BoardVO vo) {
//log.printLog();
log.printLogging();
return boardDAO.getBoardList(vo);
}
}
BoardServiceClient 를 실행하면 아래와 같은 화면을 볼 수 있다.
실행 화면 )
업그레이드 된 로그 → Log4j
여기까지는 하나하나 다 바꿔줘야 했음 → AOP가 이를 해결
로그의 기능을 이해하기 위해 예시 코드를 짜 본 것이다.
위와 같은 공통 로그를 통해 어느 부분에서 오류가 난 건지 알 수 있다.
AOP 적용하기
AOP를 사용하기 위해 라이브러리 추가가 필요하다.
<!-- AspectJ -->
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjrt</artifactId>
// ${org.aspectj-version} 버전에 맞춰 받아준다
<version>${org.aspectj-version}</version>
</dependency>
pom.wml에 AspectJ 와 AspectJ Weaver를 추가한다.
<!-- AspectJ -->
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjrt</artifactId>
<version>${org.aspectj-version}</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.aspectj/aspectjweaver -->
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjweaver</artifactId>
<version>1.8.8</version>
<scope>runtime</scope>
</dependency>
💡 스프링은 공통관심사의 대상이 메소드
applicationContext.xml 에서 )
<aop:config>
<!-- 실행 범위 -->
<aop:pointcut expression="execution(* com.springbook.biz..*Impl.*(..))" id="allPointcut"/>
<!-- 레퍼런스 log -->
<aop:aspect ref="log">
<!-- 대상, 적용할 기능 -->
<aop:before pointcut-ref="allPointcut" method="printLog"/>
</aop:aspect>
</aop:config>
"execution(* com.springbook.biz..*Impl.*(..))"
= 패키지 하위의 범위를 표현하는 정규식
= 패키지 안에 Impl 이 들어간 파일이 있다면 공통관심사 코드 실행
최종 applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.3.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.3.xsd">
<context:component-scan base-package="com.springbook.biz"></context:component-scan>
<!-- <bean id="log" class="com.springbook.biz.common.LogAdvice" ></bean> -->
<bean id="log" class="com.springbook.biz.common.Log4jAdvice" ></bean>
<aop:config>
<aop:pointcut expression="execution(* com.springbook.biz..*Impl.*(..))" id="allPointcut"/>
<aop:aspect ref="log">
<!-- 대상, 적용할 기능 -->
<aop:before pointcut-ref="allPointcut" method="printLogging"/>
</aop:aspect>
</aop:config>
</beans>
로그를 찍는 구문이 없지만 클라이언트 파일로 실행해보면 로그가 나온다.
BoardServiceImpl.java
package com.springbook.biz.board.impl;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.springbook.biz.board.BoardService;
import com.springbook.biz.board.BoardVO;
import com.springbook.biz.common.Log4jAdvice;
import com.springbook.biz.common.LogAdvice;
@Service("boardService")
public class BoardServiceImpl implements BoardService {
// boardDAO를 의존 주입해야 한다.
@Autowired
private BoardDAO boardDAO;
private Log4jAdvice log;
public BoardServiceImpl() {
}
@Override
public void insertBoard(BoardVO vo) {
boardDAO.insertBoard(vo);
}
@Override
public void updateBoard(BoardVO vo) {
boardDAO.updateBoard(vo);
}
@Override
public void deleteBoard(BoardVO vo) {
boardDAO.deleteBoard(vo);
}
@Override
public BoardVO getBoard(BoardVO vo) {
return boardDAO.getBoard(vo);
}
@Override
public List<BoardVO> getBoardList(BoardVO vo) {
return boardDAO.getBoardList(vo);
}
}
AOP의 용어들
● 조인포인트 (Joinpoint) : 공통 관심사가 적용 될 수 있는 모든 메소드들
● 포인트컷 (pointcut) : 실제 관심사가 적용되는 메소드들
● 어드바이스 (Advice) : 실제 공통 관심사
● 에스펙트 (Aspect) or 어드바이져(Adviser) : 포인트컷 + 어드바이스
- Aspect aop 적용
aop:aspect : 공통관심사 메소드가 명확할 때 사용
aop:advisor : 공통관심사 메소드가 명확하지 않을 때 (외부에서 가져다 쓰는 경우)
● 위빙 (Weaving) : 핵심관심(비즈니스 로직) 메소드에 횡단(공통) 관심사(어드바이스)가 적용되는 것.
리턴 타입 지정
리턴 타입 지정에서 가장 기본적인 방법은 ‘ * ’ 캐릭터 사용하는 것
* : 모든 리턴 타입 허용
void : 리턴 타입이 void인 메소드 선택
!void : 리턴 타입이 void가 아닌 메소드 선택
패키지 지정
페이지 경로를 지정할 때는 ‘ * ’, ‘ .. ’ 를 사용
com.springbook.biz : 정확하게 com.springbook.biz만 선택
com.springbook.biz.. : com.springbook.biz 로 시작되는 모든 패키지 선택
com.springbook..impl : impl로 끝나는 패키지 선택
어드바이스가 동작하는 시점
before : 포인트컷 실행 이전
after : 포인트컷 실행 이후
around : 포인트컷 실행 전 후
▼ around : 포인트컷 실행 전 후
코드 )
AroundAdvice.java
package com.springbook.biz.common;
import org.aspectj.lang.ProceedingJoinPoint;
public class AroundAdvice {
// 매개변수를 반드시 ProceedingJoinPoint로 써줘야 한다
public Object aroundLog(ProceedingJoinPoint pjp) throws Throwable {
System.out.println("[Before] 비즈니스 로직 수행 전");
// 비즈니스 로직 메소드의 동작을 감지
Object returnObj = pjp.proceed();
System.out.println("[After] 비즈니스 로직 수행 후");
return returnObj;
}
}
applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.3.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.3.xsd">
<!-- 패키지를 스캔해준다. 다 해주는 것은 아니고 표시되어진 클래스만 스캔해준다 -> 이것이 어노테이션 -->
<!-- <context:component-scan base-package="polymorphism"></context:component-scan> -->
<context:component-scan base-package="com.springbook.biz"></context:component-scan>
<!-- <bean id="log" class="com.springbook.biz.common.LogAdvice" ></bean> -->
<bean id="log" class="com.springbook.biz.common.Log4jAdvice" ></bean>
<bean id="around" class="com.springbook.biz.common.AroundAdvice" ></bean>
<aop:config>
<aop:pointcut expression="execution(* com.springbook.biz..*Impl.*(..))" id="allPointcut"/>
<aop:aspect ref="around"> <!-- 동작시점 -->
<!-- 대상, 적용할 기능 -->
<aop:around pointcut-ref="allPointcut" method="aroundLog"/>
</aop:aspect>
</aop:config>
</beans>
클라이언트 파일을 실행 시키면 이렇게 나온 것을 확인 할 수 있다.
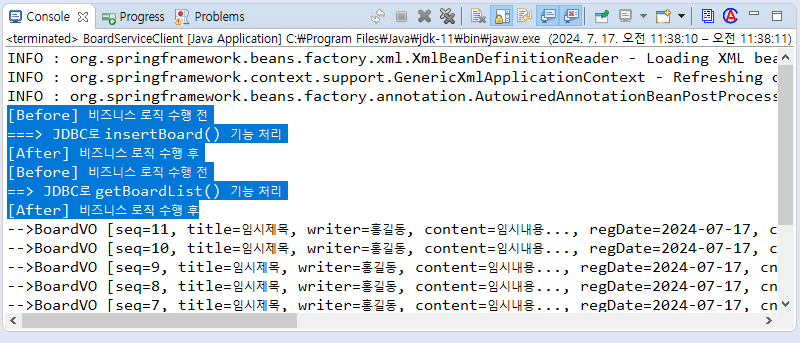
스프링 JDBC
라이브러리 다운받기
spring-jdbc
commons-dbcp
pom.xml 에 추가
<!-- https://mvnrepository.com/artifact/org.springframework/spring-jdbc -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>${org.springframework-version}</version>
</dependency>
<!-- https://mvnrepository.com/artifact/commons-dbcp/commons-dbcp -->
<dependency>
<groupId>commons-dbcp</groupId>
<artifactId>commons-dbcp</artifactId>
<version>1.4</version>
</dependency>
JdbcTemplate 객체 기능
- update()
→ insert, update, delete
- queryForInt()
- queryForObject()
- query()
-> select
'2024_UIUX 국비 TIL' 카테고리의 다른 글
UIUX _국비과정 0719 [스프링 model2방식 웹만들기] (0) | 2024.07.30 |
---|---|
UIUX _국비과정 0718 [스프링 model1방식 웹만들기] (0) | 2024.07.30 |
UIUX _국비과정 0716 [스프링 게시판, 사용자 로그인 여부 알리기] (0) | 2024.07.29 |
UIUX _국비과정 0716 [스프링 3대 요소, 게시판 기본 구조 만들기] (0) | 2024.07.29 |
UIUX _국비과정 0715 [스프링 설치, 구조 알아보기, 스프링 3대 요소] (1) | 2024.07.29 |